Web Frameworks for Node.js: A Comparative Overview
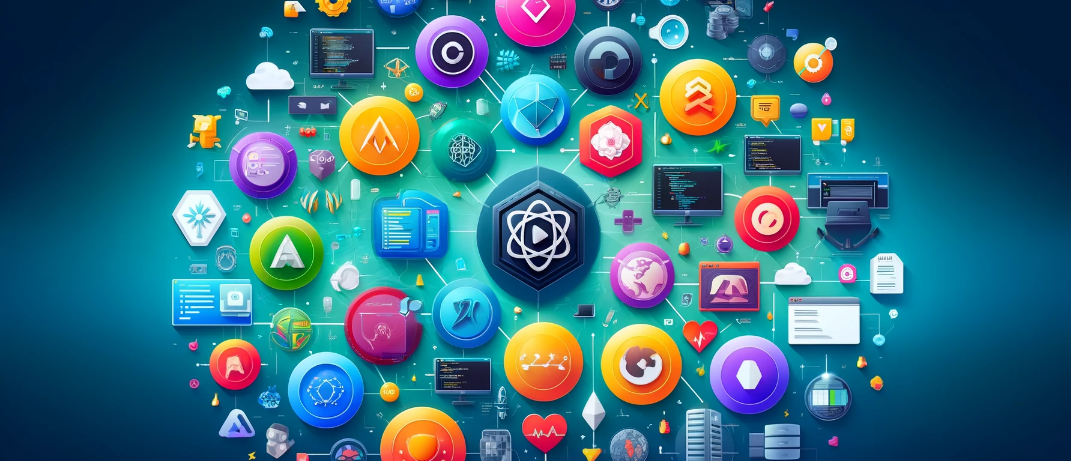
Node.js has revolutionized server-side development with its event-driven, non-blocking I/O model. Among its numerous web frameworks, some stand out due to their popularity, performance, or unique features. This article explores three top web frameworks for Node.js: Express, Fastify, and Socket.IO, along with three additional alternatives to consider for your next project.
Express
Express is the most popular, fast, and minimalist web framework for Node.js backends. It provides a robust set of features to develop web and mobile applications, making it a go-to choice for developers.
Example:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Fastify
Fastify is one of the fastest web frameworks focused on providing the best developer experience with the least overhead. It boasts a powerful plugin architecture and a high-performance HTTP server.
Example:
const fastify = require('fastify')({
logger: true
});
fastify.get('/', async (request, reply) => {
reply.type('application/json').code(200);
return { hello: 'world' };
});
fastify.listen(3000, (err, address) => {
if (err) throw err;
fastify.log.info(`Server is running on ${address}`);
});
Socket.IO
Socket.IO enables real-time, bidirectional, event-based communication using long-polling or WebSockets with disconnection detection and auto-reconnection support. It's ideal for applications requiring real-time updates.
Example:
const server = require('http').createServer();
const io = require('socket.io')(server);
io.on('connection', (client) => {
client.on('event', (data) => { /* … */ });
client.on('disconnect', () => { /* … */ });
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
Koa
Koa is a web framework designed by the creators of Express, aiming to be smaller, more expressive, and robust. It leverages async functions for enhanced error handling and cleaner code.
Example:
const Koa = require('koa');
const app = new Koa();
app.use(async ctx => {
ctx.body = 'Hello World';
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
NestJS
NestJS is a progressive Node.js framework for building efficient, reliable, and scalable server-side applications. It uses TypeScript by default and combines elements of OOP (Object-Oriented Programming), FP (Functional Programming), and FRP (Functional Reactive Programming).
Example:
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
await app.listen(3000);
}
bootstrap();
Hapi
Hapi.js is a rich framework for building applications and services. Known for its powerful plugin system, Hapi.js simplifies the development of complex applications with a high degree of customization.
Example:
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = Hapi.server({
port: 3000,
host: 'localhost'
});
server.route({
method: 'GET',
path: '/',
handler: (request, h) => {
return 'Hello World';
}
});
await server.start();
console.log('Server is running on port 3000');
};
init();
Conclusion
Choosing the right web framework for your Node.js application depends on your specific needs. The Express Node.js web application framework remains a versatile and straightforward choice, Fastify offers performance and developer-friendliness, and Socket.IO excels in real-time applications. Koa, NestJS, and Hapi provide additional robust options with unique strengths, from minimalism and modern design to enterprise-grade features. Evaluate your project requirements and consider these frameworks to enhance your Node.js development experience.